My garage door is controlled my a Lynx 455 Plus garage opener
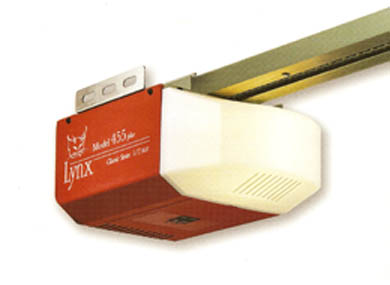
This is a pretty traditional opener; a door-bell type button inside the garage to open/close the door and a remote control for wireless access.
I wanted to see if I could make this smart-enabled. Now the control side is simple enough; just put a relay in parallel to the button. If the relay closes then the opener will think the button has been pressed.
It would also be nice to have a sensor to detect if the door is open or not. For this a magnetic reed switch would work. Mount the switch to the frame and stick the magnet to the door. When the door is closed the switch activates (e.g. closes or opens, depending on if the switch is “NC - normally closed” or “NO - normally open”) and when the door opens the magnet moves away from the reed switch and the state changes.
So the concept is easy, but what we can use to put all this together?
Enter the Shelly 1.
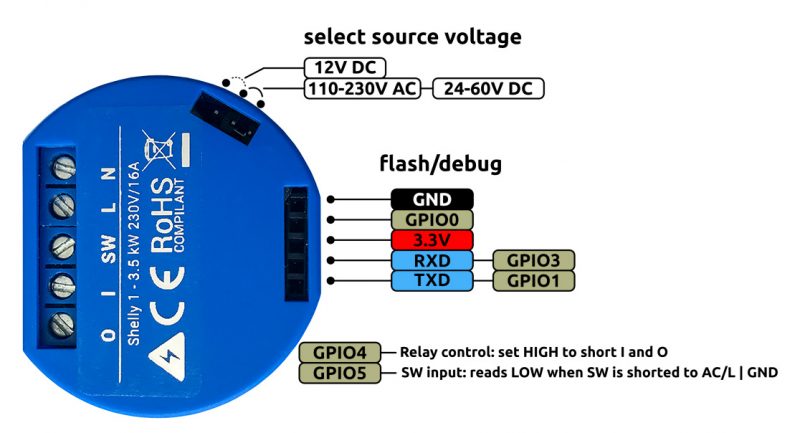
This is a small device. In some respects it’s a competitor to Sonoff switches but has some nice features. In particular it has a “switch sense” line. By default this controls the relay, but in the latest firmware that can be disconnected and acts purely as a sensor. Nicely this device can also be powered from low voltage DC and the garage door opener has 24V DC available, so this looked perfect for my needs.
Spoiler: it turned out that the 24V from the opener wasn’t stable enough to drive this, so I had to run a power line anyway. But everything else went to plan!
For Sonoff fans, the Shelly 1 can be flashed with Tasmota firmware, but I didn’t feel the need. The latest firmware did everything I need out of the box.
The Shelly 1 can also be connected to a cloud for remote control, but I didn’t want this so I never enabled it. Indeed I wanted other functionality (MQTT) which prevents cloud action. So all control is local.
Setting up the Shelly 1
On first powerup the Shelly works as a WiFi access point. From here connect a device (I used a Chromebook, but a phone would work), browse to the configuration page, tell it to become a WiFi client of your normal WiFi network. And that’s all it takes to make it reachable. I also added a username/password requirement. It’s only “basic auth” over a http connection, so it’s not very secure, but at least it stops accidents.
For the garage door opener use case I configured the “Settings” page so that on power-up the device defaults to OFF. I set the button type to “Detached switch”.
On the “Internet and Security” tab, under advanced settings I configured MQTT to point to an MQTT server on my HA machine. Note that the server has to be specified by IP address (eg 10.20.30.40:1883) and can not be SSL enabled. Fortunaly the common “mosquito” server works just fine, and is common in HA setups.
In case it helps, I also set up the following:
Min reconnect timeout: 2
Max reconnect timeout: 60
Keep alive: 60
Clean Session: y
Retain: n
Max QoS: 0
Will Topic: shellies/shelly-garage/online
Will Message: true
On the “Timer” tab I set “Auto Off: When ON Turn off after 2 seconds”. What this means is that when the relay is triggered then it will auto turn off after 2 seconds, so it acts like a press and release of the button. At least that’s how it will look to the door opener!
Monitor and control
Now with this running we should be able to see regular output messages on the MQTT server. The exact queue name will depend on the device ID. In my case I can see
% mqttcli sub -d --host hass -t shellies/shelly1-24DCEF/input/0
INFO[0000] Broker URI: tcp://hass:1883
INFO[0000] Topic: shellies/shelly1-24DCEF/input/0
INFO[0000] connecting...
INFO[0000] client connected
INFO[0003] topic:shellies/shelly1-24DCEF/input/0 / msg:1
1
INFO[0008] topic:shellies/shelly1-24DCEF/input/0 / msg:1
1
INFO[0013] topic:shellies/shelly1-24DCEF/input/0 / msg:1
1
So every 5 seconds there’s a message showing the state of the switch. In this case the switch is closed. If the switch was open then it would show a 0. Interestingly a state change causes a message to be sent immediately.
We can also control the relay by sending a message to the API
curl -X POST -u user:pass http://shelly-garage/relay/0 -d 'turn=on'
(“shelly-garage” is the DNS name I set for the Shelly). This should turn the relay on (you’ll hear it click) and then the timer should auto-turn it off after 2 seconds.
It’s also possible to control the relay by publishing to an MQTT queue, but I didn’t do that.
Alexa integration
Now we’re in a position to start controlling things!
Because I wanted to control this from Alexa, I used my fake Hue software to make it pretend to be a bulb.
Reading the switch status was done with a background process:
#!/bin/ksh -p
# ON means Garage is open
STATUS=${1:-/tmp/garage}
temp=0
mqttcli sub --host hass -t shellies/shelly1-24DCEF/input/0 | while read line
do
kill -0 $PPID 2>/dev/null || exit
if [ $line == 1 ]
then
onoff=OFF
else
onoff=ON
fi
print $onoff > $STATUS
done
I did it this way so the main loop just needs to read the /tmp/garage
file
to get the state, with no delays.
Now there’s no true open/close control for this door; you just press the
button. So for simplicity I just make on/off call the same function.
The routines used in the media
loop look like this:
get_garage()
{
SWITCH["GarageDoor"]=$(cat $GARAGE_STATUS)
}
set_garage()
{
curl --max-time 2 -X POST -u user:pass http://shelly-garage/relay/0 -d 'turn=on'
}
With this fake switch discovered by Alexa I could now say “alexa turn garagedoor on” and the door opens. Similarly “alexa turn garagedoor off”.
It’s easy to create a routine now: “Alexa open the garage door” and even “Alexa open the pod bay doors” just calls the smart home bulb on function.
Monitor and alert from Home Assistant
I thought it would be nice to have an alert whenever the door was opened or closed. Enter HA.
First we need to set up a sensor to read the state of the switch:
sensor:
- platform: mqtt
name: "Garage Door"
state_topic: "shellies/shelly1-24DCEF/input/0"
value_template: >
{% if value == "1" %}
closed
{% else %}
open
{% endif %}
This creates a sensor called sensor.garage_door
and has a state of
“open” or “closed”. In my case my reed switch returns a “1” when the
door is closed. If the switch you use returns a “0” then just change
this line.
With this we can add a badge to the standard Lovelace UI
- sensor.garage_door
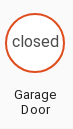
An automation can now be triggered on the change of state of the door
- alias: Detect Garage
initial_state: true
trigger:
platform: state
entity_id: sensor.garage_door
action:
service: notify.alexa_media
data_template:
target:
- media_player.living_room
- media_player.kitchen
- media_player.stairs
data:
type: announce
message: >
{% if trigger.to_state.state == "closed" %}
"Garage Door Closed"
{% else %}
"Garage Door Opened"
{% endif %}
This makes use of the alexa_media
component.
Physically setting it up.
All the work, so far, can be done on a bench.
Basically the wiring is as follows:
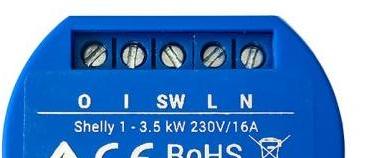
The 0/1 lines should be connected, in parallel, to the existing button push. It doesn’t matter what way round the wires go.
The SW and L lines should be connected to the reed switch. Again it doesn’t matter what way round the wires go.
Finally the L and N should be connected to the power. Since we’re using low power DC the L should be connected to ground and the N connected to the + voltage. This sounds backwards, but it’s not.
My original intent was to use the 24V power line from the door opener, but when I tried the Shelly just didn’t power up at all. So in the end I used a 12V power supply; cut the plug off it and connected the cables to the terminals.
Future state
Going forwards I would like the HA notifications to be location aware. For example if I’m away from home and the garage door is opened then ping my phone. Or if I’m leaving home and the garage door was left open then alert me. I haven’t yet found a good location tracker for HA that works with my Android phone, so that’s still a work in progress.